IOS SDK
You can use Nearpay Connect in your IOS app
Configration
-
Download the framework from here
-
Import the framework into your projects. Steps:
- In the project navigator, select your project.
- Select your target.
- Select the
General
tab. - Open "Framework , Libraries and Embedded Content" expander.
- Click
+
button. - Add file
optional
or you can just drag and drop the file to "Framework , Libraries and Embedded Content".
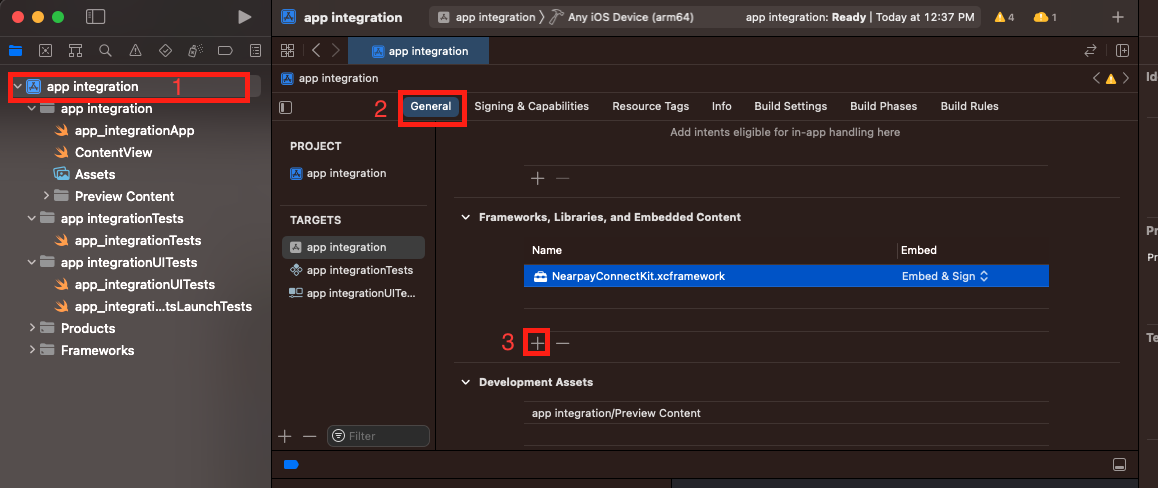
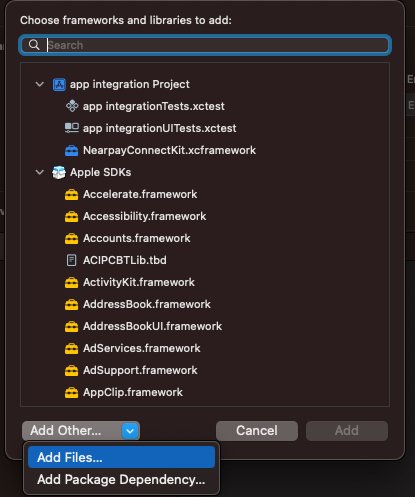
- Add bluetooth as
Bluetooth Always Usage Description
to your info.
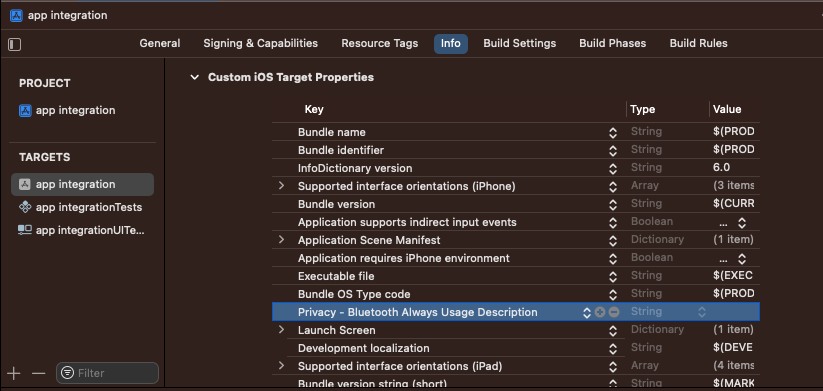
- import NearpayConnectKit
import NearpayConnectKit
- Define connectivity
object @StateObject var conn: Connectivity = Connectivity.shared
- add this modifier in the end of root view
.nearpayConnectivity()
- Add sessionDelegate in main root
.onAppear {
conn.sessionDelegate = self
}
- Start Purchase
conn.startPurchaseTransaction(amount: 300)
- Add extension to root view
extension ContentView: TransactionSessionDelegate
{
func transactionSesison(didReciviedDataFor transaction: NearpayConnectKit.RemoteTransaction?, withError error: Error?) {
print("data received \(transaction)")
}
func transactionSession(didEndWithFor transaction: NearpayConnectKit.RemoteTransaction?, withError error: Error?) {
print("data error \(error)")
}
}
Functions
This function is used to show the setupUI of Nearpay connect
conn.setupUI()
Connect the device via IP address
Start Purchase Transaction
Purchase is used to send the amount to the other Device
conn.startPurchaseTransaction(amount: 300)
Reset Setting
Reset setting is used to reset all the settings .
conn.resetSetting()
Show UI
ShowUI is a Boolean to check if UI is opened or closed.
var uiStatus = conn.showUI // it will be true or false
Example
import SwiftUI
import NearpayConnectKit
struct ContentView: View {
@StateObject var connecetivity : Connectivity = .shared
var body: some View {
VStack(spacing: 30) {
Image(systemName: "globe")
.imageScale(.large)
.foregroundColor(.accentColor)
.onTapGesture {
connecetivity.startPurchaseTransaction(amount: 300)
}
Button("settings")
{
connecetivity.setupUI()
}
Text("Hello, world! \(Connectivity.name)")
}
.padding()
.nearpayConnectivity()
.onAppear
{
connecetivity.sessionDelegate = self
}
}
}
extension ContentView: TransactionSessionDelegate
{
public func transactionSesison(didReciviedDataFor transaction: NearpayConnectKit.RemoteTransaction?, withError error: Error?) {
print("data received: \(transaction)")
}
public func transactionSession(didEndWithFor transaction: NearpayConnectKit.RemoteTransaction?, withError error: Error?) {
print("error received: \(transaction)")
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}